TypeScript Best Practices for Modern Web Development
Learn how to write clean, maintainable TypeScript code with these best practices and patterns.
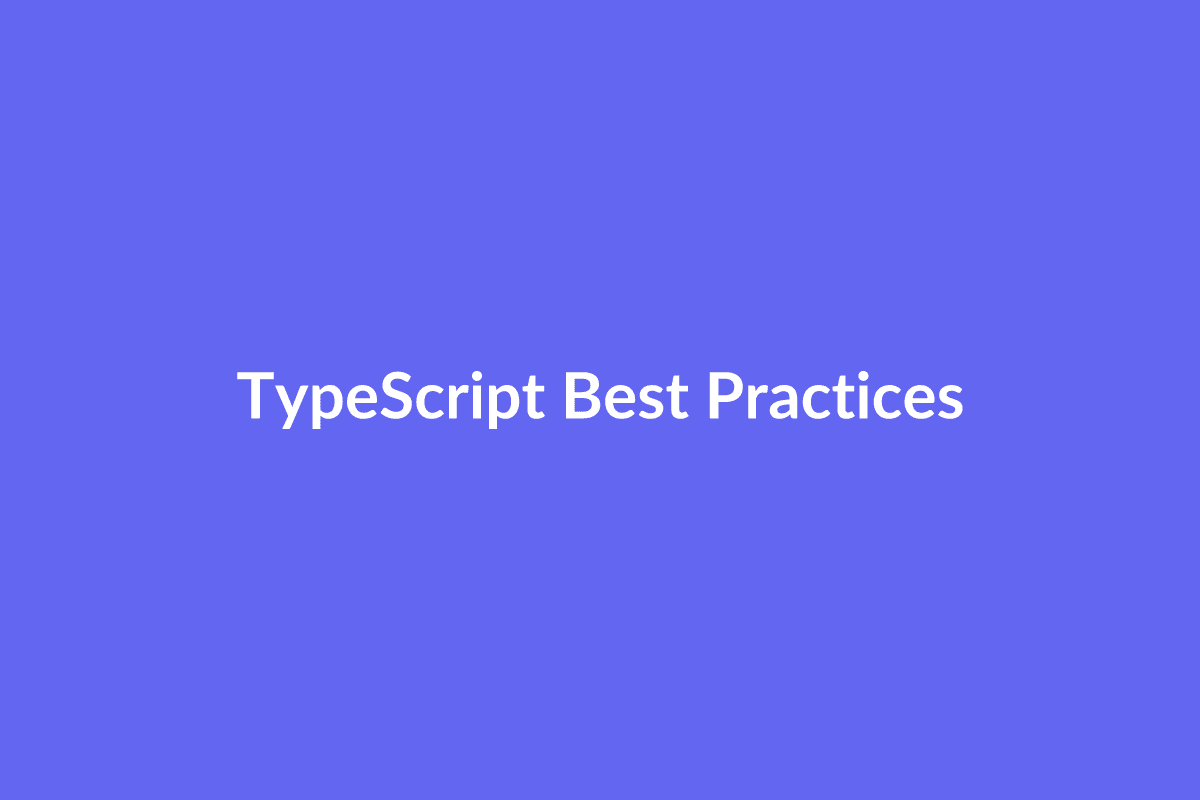
TypeScript Best Practices for Modern Web Development
TypeScript has become the language of choice for many web developers due to its strong typing system and enhanced tooling. In this post, we'll explore some best practices for writing clean, maintainable TypeScript code.
Why TypeScript?
TypeScript offers several advantages over plain JavaScript:
- Static Typing: Catch errors at compile time rather than runtime.
- Enhanced IDE Support: Better autocomplete, navigation, and refactoring tools.
- Better Documentation: Types serve as documentation for your code.
- Safer Refactoring: The compiler catches errors when you change your code.
- Modern JavaScript Features: Use the latest JavaScript features with backward compatibility.
Best Practices
1. Use Strict Mode
Enable strict mode in your tsconfig.json
to catch more potential errors:
{
"compilerOptions": {
"strict": true
}
}
2. Prefer Interfaces for Object Shapes
Use interfaces to define the shape of objects:
// Good
interface User {
id: string;
name: string;
email: string;
}
// Avoid
type User = {
id: string;
name: string;
email: string;
};
3. Use Type Inference When Possible
Let TypeScript infer types when it's clear what they should be:
// Good
const numbers = [1, 2, 3]; // TypeScript infers number[]
// Unnecessary
const numbers: number[] = [1, 2, 3];
4. Avoid Any
The any
type defeats the purpose of using TypeScript. Use more specific types or unknown
if necessary:
// Avoid
function processData(data: any) {
// ...
}
// Better
function processData(data: unknown) {
if (typeof data === 'string') {
// Now TypeScript knows data is a string
}
}
5. Use Function Types
Define function types for better documentation and type checking:
type FilterFunction<T> = (item: T) => boolean;
function filter<T>(array: T[], predicate: FilterFunction<T>): T[] {
return array.filter(predicate);
}
Conclusion
TypeScript is a powerful tool for building robust web applications. By following these best practices, you can write cleaner, more maintainable code that's less prone to bugs and easier to refactor.